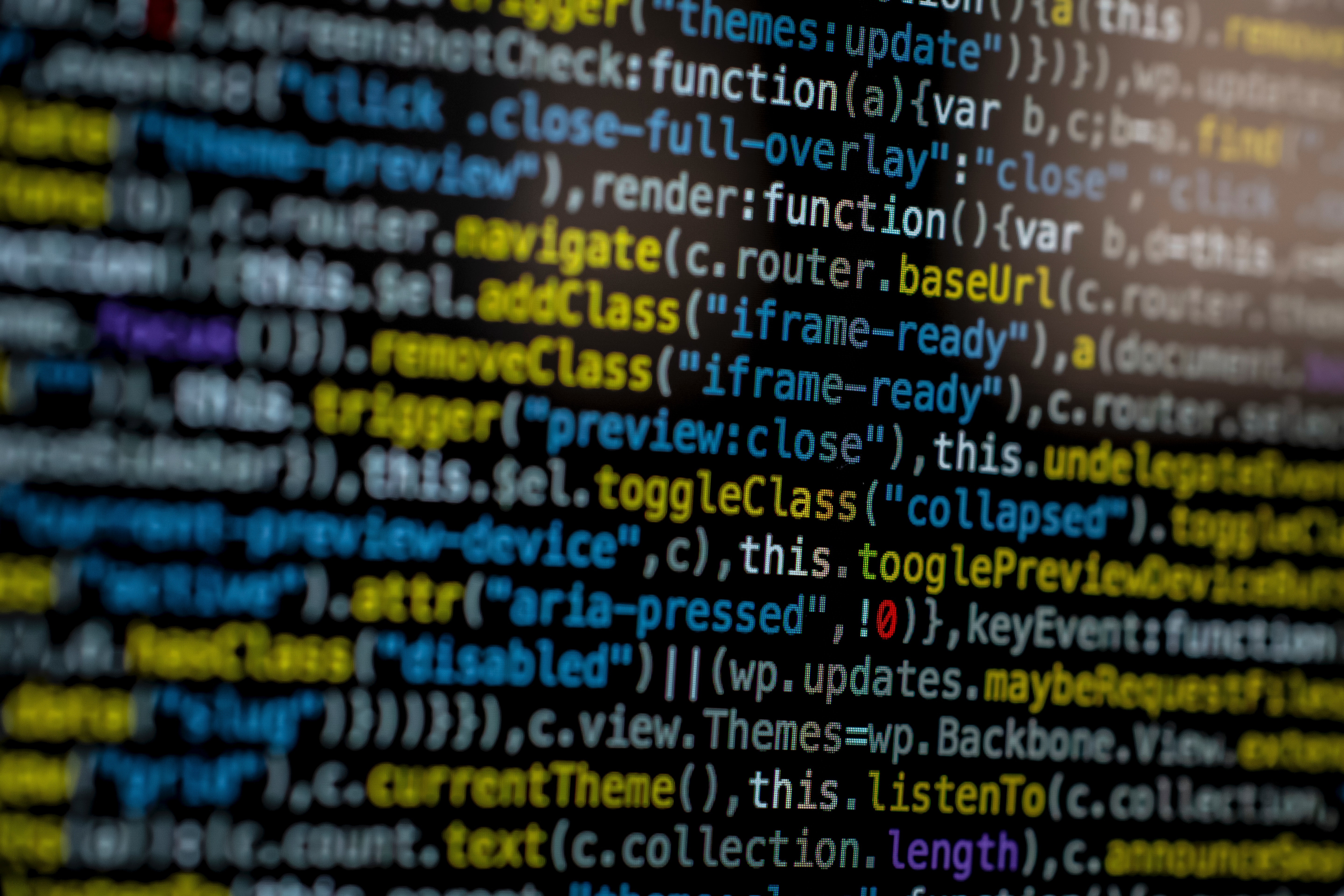
JavaScript is currently the most in-demand language. If you are looking for one language to learn in 2019 then JavaScript is definitely the one. There are different frameworks which made JavaScript as popular as it is now, which includes React.js, Angular.js.
So throught this series we will be looking at some Advanced JavaScript concepts. Today’s topic is Function Constructors.
Let’s now create a Function Constructor in JavaScript:
let Person = function (name, age, sex) {
//New Object being created and initialized
this.name = name;
this.age = age;
this.sex = sex;
}
So, Person here is a Constructor and we always write Function Constructors with a capital letter.
However, this used to be the older approach of creating Function Constructor, but it still works on the newer version on JavaScript.
Here’s the ES2015 way of creating Function Constructor
//Name of ES5 Constructor
class Person {
//New Object being created and initialized
constructor(name, age, sex) {
this.name = name;
this.age = age;
this.sex = sex;
}
}
Now lets create a new Object. Let’s make it Buddy object and lets display the output on console.
let Buddy = new Person('Buddy1', 22, 'Male');
console.log(Buddy);
Here, as per the approach is shown above we are creating new objects using Function Constructors. We are creating a new Buddy Object and passing arguments into the Function. The Buddy object here is the instances of the Person object.
So we are creating new objects using Function Constructor rather than making different objects using object literal which would had been hard and cause issues and be repeating the code too much.
Here’s the output of the program :

Next up we will be discussing over the Prototype Chain